Editorial
First, let's consider an operation that is the inverse of .
Let be represented by a bit string .
Based on this representation, the operation can be rephrased as follows:
- Convert all consecutive 's from the least significant bit to and flip the first bit to .
- Specifically, find such that "" and flip to and to . If "", flip to . If "", flip to .
Let's consider the example of below.
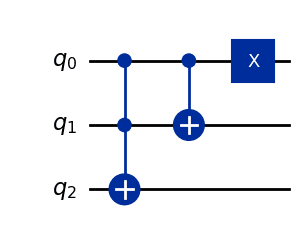
Assume that the input state is .
First, the state transition is as follows by the "multi-controlled gate1" with the first and second qubits as control bits and the third qubit as the target bit.
Next, the state transition is as follows by the "controlled gate with the first qubit as the control bit and the second qubit as the target bit".
Finally, the state transition is as follows by the gate.
As a result, the operation is realized.
By generalizing the above operation, the operation can be realized, and the code is as follows:
from qiskit import QuantumCircuit
def solve(n: int) -> QuantumCircuit:
qc = QuantumCircuit(n)
for i in reversed(range(1, n)):
qc.mcx(list(range(i)), i)
qc.x(0)
return qc
By applying these gates in reverse order, the operation is realized.
Sample Code
Below is a sample program:
from qiskit import QuantumCircuit
def solve(n: int) -> QuantumCircuit:
qc = QuantumCircuit(n)
qc.x(0)
for i in range(1, n):
qc.mcx(list(range(i)), i)
return qc
Equivalently, you can use the inverse method.
from qiskit import QuantumCircuit
def solve(n: int) -> QuantumCircuit:
qc = QuantumCircuit(n)
for i in reversed(range(1, n)):
qc.mcx(list(range(i)), i)
qc.x(0)
return qc.inverse()
Follow-up
By decomposing a positive integer into the sum of and , the algorithm of this problem can realize by applying the algorithm at most times.