Q&A
Last updated: October 25th, 2024.
Contents
- About QCoder
- What is QCoder?
- I'm unfamiliar with quantum computing. What should I do?
- How can I implement quantum algorithms?
- I want to study quantum computing. Do you have any recommended materials?
- I want to know about upcoming contests and updates.
- What kind of submission statuses are there?
- What libraries and modules can be used?
- What quantum gates can be used?
- Can I add extra qubits to the circuit?
- Can I execute quantum circuits on my local computer?
- What is a Python compile error?
- What are the versions of Python and of the libraries?
- I am interested in QCoder's activities. How can I contribute to creating problems or operations?
- About Quantum Computing
About QCoder
What is QCoder?
QCoder is a new platform for quantum competitive programming launched in 2024.
Unlike traditional competitive programming contests, QCoder presents problems that require the implementation of quantum algorithms that run on quantum computers.
I'm unfamiliar with quantum computing. What should I do?
We recommend starting by participating in the contest.
While you might find many parts confusing at first, you'll gradually understand the bigger picture as you solve problems and refer to the editorial provided.
QCoder aims to offer contests that can be enjoyed by everyone, from those who know nothing about quantum computing to experts.
How can I implement quantum algorithms?
Currently, we support the use of Qiskit, a quantum programming language (framework) that operates on Python.
Submitted programs are evaluated using a simulator, not an actual quantum computer.
*The editor on QCoder does not support autocompletion or linters. We recommend setting up a Qiskit environment on your local computer.
I want to study quantum computing. Do you have any recommended materials?
The following materials are recommended:
- Basics of quantum information - IBM Quantum Learning:
Official Qiskit tutorials on quantum computing. - Qubit Factory:
A puzzle/construction game about manipulating qubits to solve challenges.
I want to know about upcoming contests and updates.
Please follow our SNS accounts and register on Google Calendar.
What kind of submission statuses are there?
The following submission results may be displayed:
- AC (Accepted): The submission passed all test cases.
- WJ (Waiting for Judging): The submission is waiting to be judged.
- WA (Wrong Answer): The submission did not pass at least one test case.
- QLE (Qubits Limit Exceeded): The number of qubits exceeded the limit.
- DLE (Depth Limit Exceeded): The circuit depth exceeded the limit.
- TLE (Time Limit Exceeded): The execution time exceeded the limit.
- MLE (Memory Limit Exceeded): The memory usage exceeded the limit.
- CE (Compilation Error): Some error occurred during the compilation.
- RE (Runtime Error): Some error occurred during the program execution.
- UME (Unauthorized Module Error): An unauthorized module was imported.
- UGE (Unauthorized Gate Error): An unauthorized quantum gate was present in the circuit.
- TOE (Time Out Error): A certain amount of time has passed without the submission being judged.
What libraries and modules can be used?
Currently, only the following Python modules are permitted for use.
If any modules other than these are imported, an UME (Unauthorized Module Error) will be displayed.
- Standard Library
- math (
import math
)
- math (
- Qiskit
- QuantumCircuit (
from qiskit import QuantumCircuit
) - QuantumRegister (
from qiskit import QuantumRegister
)
- QuantumCircuit (
- qiskit.circuit.library.standard_gates
- Any class from this module can be imported. Examples of importable classes include:
- HGate (
from qiskit.circuit.library.standard_gates import HGate
) - CXGate (
from qiskit.circuit.library.standard_gates import CXGate
) - MCPhaseGate (
from qiskit.circuit.library.standard_gates import MCPhaseGate
)
- HGate (
- Any class from this module can be imported. Examples of importable classes include:
- External library
What quantum gates can be used?
You are permitted to use quantum gates classified under Qiskit's standard gates as well as multi-control gates like the MCPhaseGate.
The use of gates that do not fall under these categories, such as the following, is prohibited and will display an UGE (Unauthorized Gate Error) if used:
Can I add extra qubits to the circuit?
You can add qubits to a quantum circuit as follows:
Open
qc = QuantumCircuit(1)
x = QuantumRegister(3)
qc.add_bits(x)
The added extra qubits must finally return to the zero state in the circuit.
Adding qubits increases the execution time. It is recommended to add only the minimum number of qubits.
Can I execute quantum circuits on my local computer?
You can execute quantum circuits that you have created on your own device.
For example, you can output the final state vector of a circuit using the following code:
Open
from qiskit import QuantumCircuit
from qiskit.quantum_info import Statevector
def solve() -> QuantumCircuit:
qc = QuantumCircuit(1)
# Write your code here:
return qc
if __name__ == "__main__":
qc = solve()
print(Statevector(qc))
What is a Python compile error?
In Python, the source file is compiled into bytecode before code execution.
This is usually done automatically at runtime, and the result is cached, but you can also manually compile using py_compile
.
In QCoder, py_compile
is performed before execution to prevent any impact on execution time.
What are the versions of Python and of the libraries?
Currently, submissions are judged on the judge server using Python 3.11.
Please refer to the requirements.lock
file below for the versions of the libraries used in the judge environment.
Open
dill==0.3.9
mpmath==1.3.0
numpy==2.1.2
pbr==6.1.0
psutil==6.1.0
python-dateutil==2.9.0.post0
qiskit==1.2.4
qiskit-aer==0.15.1
rustworkx==0.15.1
scipy==1.14.1
six==1.16.0
stevedore==5.3.0
symengine==0.13.0
sympy==1.13.3
typing_extensions==4.12.2
QCoder regularly updates the judging environment, however backward compatibility is not guaranteed.
I am interested in QCoder's activities. How can I contribute to creating problems or operations?
We are looking for people who can contribute to problem creation (Writer) and operations, as well as sponsoring companies.
If you are interested, please feel free to contact us through the contact link.
About Quantum Computing
What is a Quantum State?
In quantum computing, computings are performed using "quantum bits" (qubits), which are the quantum equivalent of bits in classical computing.
The arbitrary state of a single qubit can be expressed as a superposition of states and with coefficients and as follows:
In this manner, a qubit can exist in a probabilistic superposition of and , and it collapses to either or upon "measurement".
The coefficients and are complex numbers called "probability amplitudes". When is measured, state is observed with probability , and state is observed with probability .
Since one of the states will always be observed upon measurement, the probability amplitudes and satisfy the normalization condition .
This state of the qubit is referred to as a "quantum state".
Additionally, if we represent the states and as vectors like:
then the quantum state can be represented as:
This vector representation is known as the "state vector" of the quantum state.
Next, let's consider the quantum state of multiple qubits.
The arbitrary quantum state of qubits can be expressed as a superposition of states as follows:
As with the single-qubit case, the normalization condition holds.
For example, the quantum states of 2 qubits include , , or .
What is a Computational Basis State?
A computational basis state refers to a state of the form , where . Examples of computational basis states are: , or .
For example, the quantum state of 2 qubits
is a uniform superposition of the 2-qubit computational basis states , , , and .
Computational basis states can also be represented using decimal notation instead of binary notation, as in .
What is a Quantum Gate?
In quantum computing, the concept of a "quantum gate" is used to manipulate quantum states.
For example, a quantum gate called " gate" inverts the computational basis state of a qubit.
Besides the gate, there are many other types of quantum gates.
Understanding the meaning and role of each quantum gate may be helpful in solving future problems.
What is an Oracle?
An oracle is a term used in quantum algorithms to refer to some operation treated as a black box.
By defining the interface (input and output) without considering the implementation of the oracle as a black box, the complexity of the upstream quantum algorithm can be evaluated by the number of oracle calls.
However, the oracle itself must also be implemented as a quantum circuit.
By defining some function with input and output as computational basis states, the oracle can be defined as follows:
- When the input and output are the same state:
- When the input and output are different states:
Note that when applying the oracle, the function acts on all computational basis states appearing in the decomposition of the target quantum state.
Please also refer to the following web pages:
What is a Circuit Depth?
In quantum circuits, multiple quantum gates are stacked on each qubit in a manner similar to Tetris, describing "which quantum gate acts on which qubit in what order."
The circuit depth refers to the number of layers of quantum gates stacked in this manner.
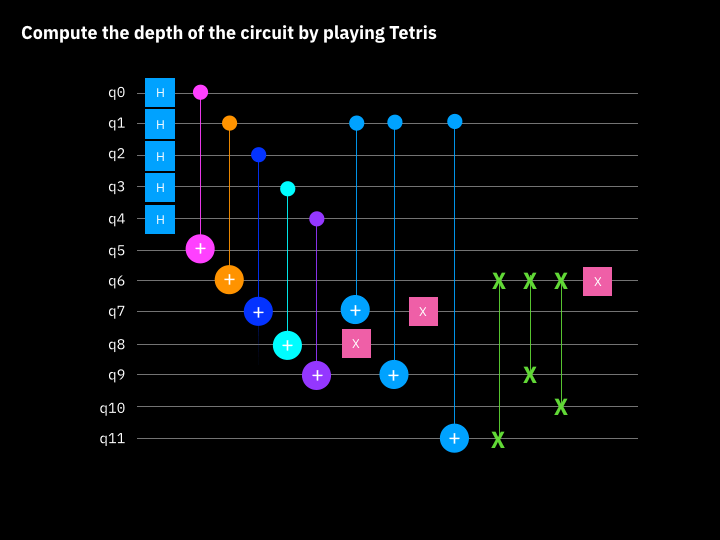
(From Quantum Circuits - IBM Quantum Documentation)
Executing a quantum circuit can be thought of as applying these layers sequentially to the qubits, which can be interpreted as a metric similar to the time complexity of classical algorithms.
In QCoder, the return value of the depth
function of Qiskit's QuantumCircuit class is treated as the depth of the circuit.
What is a Global Phase?
Consider a quantum state and a quantum state obtained by multiplying it by , where is the imaginary unit and is some real number.
Here, and are said to be equal quantum states except for the global phase .
For example, and are considered equal except for a global phase .
Since the global phase does not affect the measurement results of the quantum state, quantum states with different global phases are judged to be equivalent in QCoder.