Editorial
This problem can be solved by replacing the initial Hadamard gates in the solution for Problem B6 with a quantum Fourier transform.
The circuit diagram for the case where and is shown as follows.
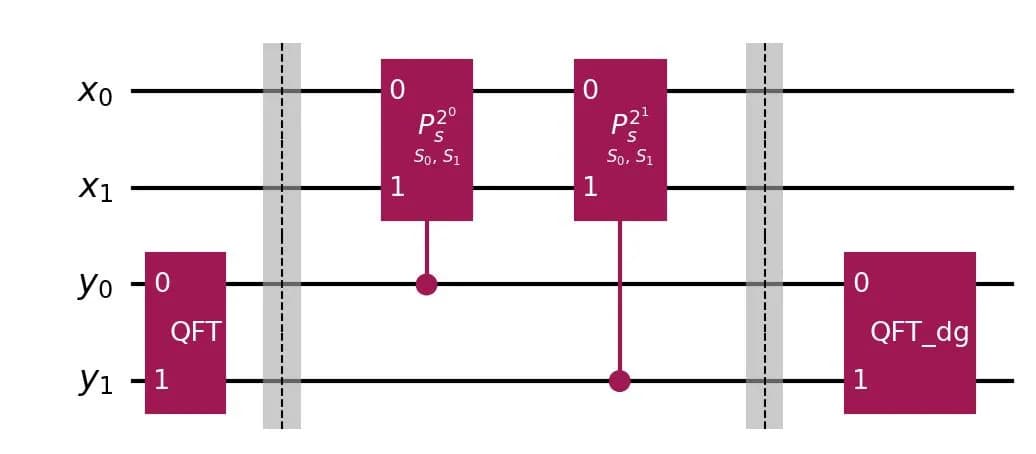
Let's examine the state transitions of the computational basis state .
First, we apply the quantum Fourier transform to the register .
Next, we apply the oracles defined in the editorial for Problem B6 using the -th qubit of the register as the control qubit.
Finally, by applying the inverse quantum Fourier transform, we can transition the register to the desired state.
Through these operations, we can implement a quantum circuit that realizes the state transition expressed as
The circuit depth is .
Sample Code
Below is a sample program:
import math
from qiskit import QuantumCircuit, QuantumRegister
def qft(n: int) -> QuantumCircuit:
qc = QuantumCircuit(n)
for i in reversed(range(n)):
qc.h(i)
for j in reversed(range(i)):
qc.cp(math.pi / 2 ** (i - j), j, i)
for i in range(n // 2):
qc.swap(i, n - i - 1)
return qc
def solve(n: int, m: int, S: list[int]) -> QuantumCircuit:
x, y = QuantumRegister(n), QuantumRegister(m)
qc = QuantumCircuit(x, y)
qft_m = qft(m)
qc.compose(qft_m, y, inplace=True)
for j in range(m):
for i in range(n):
theta = (2 * math.pi * S[i] / 2**m) * 2**j
qc.cp(theta, x[i], y[j])
qc.compose(qft_m.inverse(), y, inplace=True)
return qc